
Passing a logical vector returns the slice of the vector containing the elements where the index is TRUE.įor named vectors, passing a character vector of names returns the slice of the vector containing the elements with those names. Passing a vector of negative numbers returns the slice of the vector containing the elements everywhere except at those locations. The first position is 1 (not 0, as in some other languages). Passing a vector of positive numbers returns the slice of the vector containing the elements at those locations. If you shorten a vector, the values at the end will be removed, and if you extend a vector, missing values will be added to the end: poincare <- c ( 1, 0, 0, 0, 2, 0, 2, 0 ) #See It is also possible to assign a new length to a vector, but this is an unusual thing to do, and probably indicates bad code. For that, we should use nchar: sn <- c ( "Sheena", "leads", "Sheila", "needs" ) With these, the length is the number of strings, not the number of characters in each string. One possible source of confusion is character vectors. Missing values still count toward the length: length ( 1: 5 ) # 5 length (c ( TRUE, FALSE, NA )) # 3 This is a nonnegative integer (yes, zero-length vectors are allowed), and you can access this value with the length function. That is, all vectors have a length, which tells us how many elements they contain. I’ve just sneakily introduced a new concept related to vectors. Seq_along creates a sequence from 1 up to the length of its input: pp <- c ( "Peter", "Piper", "picked", "a", "peck", "of", "pickled", "peppers" ) for (i in seq_along (pp )) print (pp ) # "Peter"įor each of the preceding examples, you can replace seq.int, seq_len, or seq_along with plain seq and get the same answer, though there is no need to do so. However, the function is extremely useful for situations when its input could be zero: n <- 0 1:n #not what you might expect! # 1 0 seq_len (n ) # integer(0) Seq_len creates a sequence from 1 up to its input, so seq_len(5) is just a clunkier way of writing 1:5. With two inputs, it works exactly like the colon operator: seq.int ( 3, 12 ) #same as 3:12 # 3 4 5 6 7 8 9 10 11 12
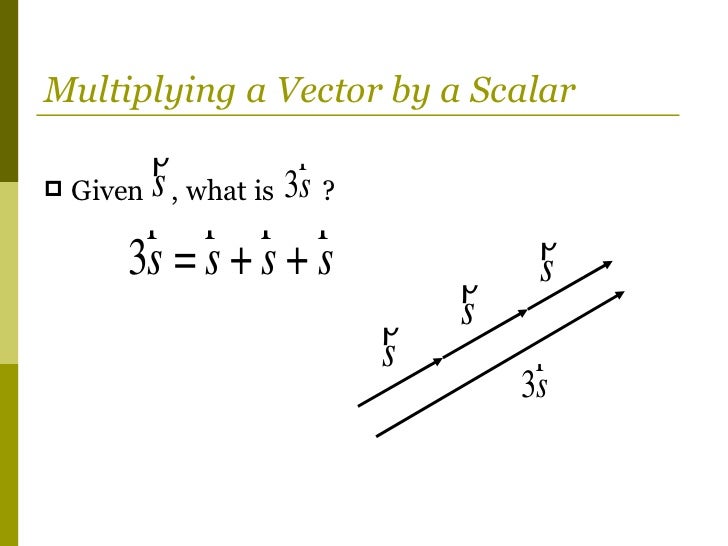
Seq.int lets us create a sequence from one number to another. In practice, though, you should never need to call it, since there are three other specialist sequence functions that are faster and easier to use, covering specific use cases. The seq function is the most general, and allows you to specify sequences in many different ways. The following commands are equivalent to the previous ones: numeric ( 5 ) # 0 0 0 0 0 complex ( 5 ) # 0+0i 0+0i 0+0i 0+0i 0+0i logical ( 5 ) # FALSE FALSE FALSE FALSE FALSE character ( 5 ) # "" "" "" "" ""īeyond the colon operator, there are several functions for creating more general sequences. For convenience, wrapper functions exist for each type to save you typing when creating vectors in this way. We’ll look at NULL in detail in Chapter 5. In that last example, NULL is a special “empty” value (not to be confused with NA, which indicates a missing data point). Each of the values in the result is zero, FALSE, or an empty string, or whatever the equivalent of “nothing” is: vector ( "numeric", 5 ) # 0 0 0 0 0 vector ( "complex", 5 ) # 0+0i 0+0i 0+0i 0+0i 0+0i vector ( "logical", 5 ) # FALSE FALSE FALSE FALSE FALSE vector ( "character", 5 ) # "" "" "" "" "" vector ( "list", 5 ) # ]


The vector function creates a vector of a specified type and length.
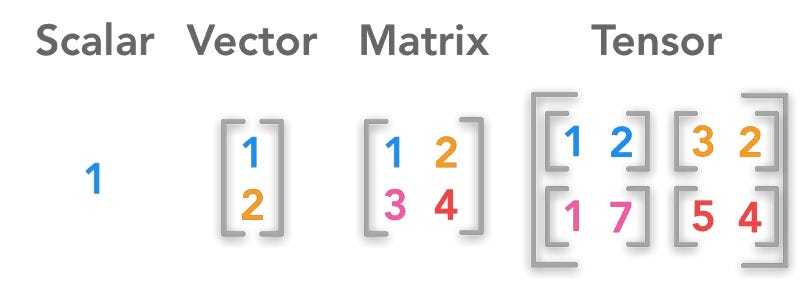
So far, you have used the colon operator, :, for creating sequences from one number to another, and the c function for concatenating values and vectors to create longer vectors.
